Composite pieces¶
Objectives¶
![]() |
In this station a figure for the halma game will be created. It is a composition from mesh objects (primitives). |
Instructions¶
Tasks: |
---|
- Create a composite pice as shown in this learning unit.
- Move the new object with the mouse.
- Join the parts and name the object »halma_figure«.
- Move the new object again.
- Duplicate the object and have a look at the counter in the header of the info window.
First version¶
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 | #! bpy
"""
Name: 'halma_figure.py'
Blender: 269
Group: 'Example composit piece'
Tooltip: 'Crate and add a halma figure'
"""
import bpy
def halma_figure():
'''Crate a halma figure from a ball and a cone'''
# get the context
scn = bpy.context.scene
# names of the parts
parts = ['head', 'body', 'halma_figure']
# crate the head
bpy.ops.mesh.primitive_uv_sphere_add(location=(2, 2, .9))
obj = bpy.context.object
obj.scale[0] = .6
obj.scale[1] = .6
obj.scale[2] = .6
obj.name = parts[0]
scn.objects.active = scn.objects[parts[0]]
# create the body
bpy.ops.mesh.primitive_cone_add(location=(2, 2, 0))
obj = bpy.context.object
obj.scale[0] = .8
obj.scale[1] = .8
obj.name = parts[1]
scn.objects.active = scn.objects[parts[1]]
if __name__ == '__main__':
bpy.ops.object.select_by_type(type='MESH')
bpy.ops.object.delete()
halma_figur()
|
Select the context¶
Selecting the context is the first step. Next step is creating and positioning objects. The context is assigned to a variable named scn.
# get the context
scn = bpy.context.scene
Name it¶
If you name the objects, later on it is easier to select one of them.
# names of the parts
parts = ['head', 'body', 'halma_figure']
First part of the composite pice¶
The sphere is the first part. We also scale the object afterwards and name it »head«.
# crate the head
bpy.ops.mesh.primitive_uv_sphere_add(location=(2, 2, .9))
obj = bpy.context.object
obj.scale[0] = .6
obj.scale[1] = .6
obj.scale[2] = .6
obj.name = parts[0]
Second part of the composite pice¶
Same procedure as with the first part.
# create the body
bpy.ops.mesh.primitive_cone_add(location=(2, 2, 0))
obj = bpy.context.object
obj.scale[0] = .8
obj.scale[1] = .8
obj.name = parts[1]
scn.objects.active = scn.objects[parts[1]]
Call the function¶
Let’s have a look to the final version. Are all pieces on the right place and have the right dimension? The old objects are deleted, so a frech and empty scene is used. You can check the info window. There are also statistics about the number of objects.
if __name__ == '__main__':
bpy.ops.object.select_by_type(type='MESH')
bpy.ops.object.delete()
halma_figur()

How many objects are selected/available?¶
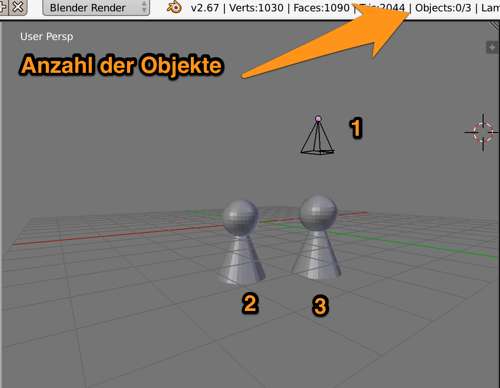
How to connect the parts to one?¶
Add the following lines of code to glue together the two pieces to a new one.
# connect parts to a new one
scn.objects[parts[0]].select = True
scn.objects[parts[1]].select = True
bpy.ops.object.join()
obj.name = parts[2]